Simulation
We can bring together the detector and source modelling to calculate the expected number of neutrino events and run simulations.
[1]:
import numpy as np
from matplotlib import pyplot as plt
from matplotlib.patches import Circle
from matplotlib.collections import PatchCollection
import h5py
Defining a source and detector model
[2]:
from icecube_tools.detector.effective_area import EffectiveArea
from icecube_tools.detector.energy_resolution import EnergyResolution
from icecube_tools.detector.angular_resolution import AngularResolution
from icecube_tools.detector.detector import IceCube
from icecube_tools.source.flux_model import PowerLawFlux
from icecube_tools.source.source_model import DiffuseSource, PointSource
from icecube_tools.detector.r2021 import R2021IRF
from icecube_tools.utils.data import SimEvents
[3]:
# Define detector (see detector model notebook for more info)
aeff = EffectiveArea.from_dataset("20210126", "IC86_II")
irf = R2021IRF.from_period("IC86_II")
# IceCube expects an instance of EffectiveAerea, AngularResolution and
# EnergyResolution, optionally the period (here IC86_I)
# R2021IRF inherits from AngularResolution and EnergyResolution
# just to be able to be used as both
detector = IceCube(aeff, irf, irf, "IC86_II")
[4]:
# Define simple sources (see source model notebook for more info)
diff_flux_norm = 3e-21 # Flux normalisation in units of GeV^-1 cm^-2 s^-1 sr^-1
point_flux_norm = 5e-19 # Flux normalisation in units of GeV^-1 cm^-2 s^-1
norm_energy = 1e5 # Energy of normalisation in units of GeV
min_energy = 1e2 # GeV
max_energy = 1e8 # GeV
diff_power_law = PowerLawFlux(diff_flux_norm, norm_energy, 3.7, min_energy, max_energy)
diff_source = DiffuseSource(diff_power_law, z=0.0)
point_power_law = PowerLawFlux(
point_flux_norm, norm_energy, 2.5, min_energy, max_energy
)
point_source = PointSource(point_power_law, z=0.0, coord=(np.pi, np.deg2rad(30)))
sources = [diff_source, point_source]
Expected number of neutrino events
Sometimes we just want to predict the number of events from sources in a detector without specifying all detector properties or running a simulation. We can do this with the NeutrinoCalculator
. For this, we just need a source list and an effective area.
[5]:
from icecube_tools.neutrino_calculator import NeutrinoCalculator, PhiSolver
[6]:
nu_calc = NeutrinoCalculator(sources, aeff)
nu_calc(
time=1, # years
min_energy=min_energy,
max_energy=max_energy, # energy range
min_cosz=-1,
max_cosz=1,
) # cos(zenith) range
[6]:
[185.18577999134666, 24.51408397397894]
The calculator returns a list of expected neutrino event numbers, one for each source.
We may also want to do the inverse, and find the PointSource
flux normalisation corresponding to an expected number of events. For this there is the PhiSolver
class.
[7]:
phi_solver = PhiSolver(
aeff, norm_energy, min_energy, max_energy, time=1, min_cosz=-1, max_cosz=1
)
phi_norm = phi_solver(Nnu=15, dec=-30, index=2.0) # degrees # spectral index
phi_norm # GeV^-1 cm^-2 s^-1
/opt/hostedtoolcache/Python/3.9.18/x64/lib/python3.9/site-packages/icecube_tools/neutrino_calculator.py:294: RuntimeWarning: The iteration is not making good progress, as measured by the
improvement from the last ten iterations.
phi_norm = fsolve(self._solve_for_phi, x0=guess, args=(Nnu, dec, index))[0]
[7]:
1.1444338237452659e-17
Set up and run simulation
[8]:
from icecube_tools.simulator import Simulator, TimeDependentSimulator
[9]:
# Set up simulation
simulator = Simulator(sources, detector, "IC86_II")
simulator.time = 1 # year
# Run simulation
simulator.run(show_progress=True, seed=42)
- Sampling: 0%| | 0/204 [00:00<?, ?it/s]
</pre>
- Sampling: 0%| | 0/204 [00:00<?, ?it/s]
end{sphinxVerbatim}
Sampling: 0%| | 0/204 [00:00<?, ?it/s]
- Sampling: 7%|▋ | 15/204 [00:00<00:02, 81.12it/s]
</pre>
- Sampling: 7%|▋ | 15/204 [00:00<00:02, 81.12it/s]
end{sphinxVerbatim}
Sampling: 7%|▋ | 15/204 [00:00<00:02, 81.12it/s]
- Sampling: 20%|██ | 41/204 [00:00<00:01, 153.38it/s]
</pre>
- Sampling: 20%|██ | 41/204 [00:00<00:01, 153.38it/s]
end{sphinxVerbatim}
Sampling: 20%|██ | 41/204 [00:00<00:01, 153.38it/s]
- Sampling: 37%|███▋ | 76/204 [00:00<00:00, 215.04it/s]
</pre>
- Sampling: 37%|███▋ | 76/204 [00:00<00:00, 215.04it/s]
end{sphinxVerbatim}
Sampling: 37%|███▋ | 76/204 [00:00<00:00, 215.04it/s]
- Sampling: 79%|███████▉ | 162/204 [00:00<00:00, 427.43it/s]
</pre>
- Sampling: 79%|███████▉ | 162/204 [00:00<00:00, 427.43it/s]
end{sphinxVerbatim}
Sampling: 79%|███████▉ | 162/204 [00:00<00:00, 427.43it/s]
- Sampling: 100%|██████████| 204/204 [00:00<00:00, 237.37it/s]
</pre>
- Sampling: 100%|██████████| 204/204 [00:00<00:00, 237.37it/s]
end{sphinxVerbatim}
Sampling: 100%|██████████| 204/204 [00:00<00:00, 237.37it/s]
This way, the simulator calculates the expected number of neutrinos from these sources given the observation period, effective area and relevant source properties. We note that we could also run a simulation for a fixed number of neutrinos if we want, simply by passing the optional argument N
to simulator.run()
.
[10]:
simulator.write_to_h5("h5_test.hdf5", sources)
[11]:
simulator.arrival_energy
[11]:
{'IC86_II': array([7.14210028e+02, 3.23388666e+02, 1.80111166e+03, 1.98115885e+02,
2.30965248e+02, 1.72795141e+02, 5.17489092e+03, 4.40330898e+02,
1.55148412e+03, 2.65048351e+02, 1.58823383e+02, 1.15940566e+03,
1.51603933e+02, 1.75155020e+02, 1.03268577e+02, 4.28410295e+02,
8.98147427e+02, 7.58773870e+02, 6.71234648e+02, 2.58801769e+03,
3.14632116e+02, 5.38505911e+02, 1.62723177e+03, 2.67854713e+02,
2.70530741e+02, 9.34743516e+02, 1.81460670e+02, 5.40258039e+02,
6.55468755e+02, 5.75003650e+02, 1.26075824e+02, 4.85847409e+02,
2.82589224e+02, 7.96531291e+02, 4.12075792e+02, 3.12642591e+02,
1.65938649e+03, 6.77278871e+02, 4.99813029e+02, 6.13118787e+02,
3.37696378e+02, 7.65201578e+02, 5.92951977e+02, 1.23201044e+02,
1.89469848e+02, 1.13214053e+03, 4.72584760e+02, 1.31327456e+02,
1.02745485e+03, 4.91791232e+02, 9.44514953e+02, 2.87311325e+03,
8.21048861e+02, 3.91184938e+02, 4.30137055e+02, 5.29922706e+02,
4.00587635e+02, 5.29919795e+02, 8.76724899e+02, 1.48299500e+02,
5.72771988e+02, 8.36602314e+02, 1.14681697e+04, 2.44752024e+02,
3.22245652e+02, 1.02005339e+03, 3.18125764e+02, 1.66246099e+03,
8.74941849e+02, 1.36462011e+03, 1.07369025e+03, 2.70848742e+02,
3.18249906e+02, 1.71764214e+03, 4.08723343e+03, 3.58361899e+02,
1.37478269e+02, 2.97152214e+02, 1.94141437e+02, 5.22309151e+02,
1.83344084e+02, 2.05133385e+02, 1.17666210e+03, 1.75173781e+03,
4.17638181e+02, 2.93957666e+02, 5.93200498e+02, 1.75312242e+02,
1.83150316e+02, 1.06684211e+03, 1.72164010e+02, 1.35537496e+03,
5.13959376e+02, 1.70974415e+03, 6.70479213e+02, 7.40572983e+02,
1.10229533e+02, 2.99456764e+03, 6.86612595e+02, 2.55393545e+03,
3.72667504e+02, 1.29488188e+02, 6.31630310e+02, 1.02579774e+03,
3.29540598e+02, 4.25228671e+02, 6.77594815e+02, 4.44957907e+02,
6.97754095e+03, 2.37937436e+02, 1.28082738e+03, 1.41709525e+02,
3.98169839e+02, 4.05308978e+02, 3.46877306e+02, 1.57425592e+02,
1.98626686e+02, 2.97095507e+02, 1.20618178e+02, 1.01980968e+02,
6.20139953e+02, 3.19540816e+02, 2.10449500e+02, 1.13580156e+03,
8.41845940e+02, 1.96038638e+02, 1.06209981e+03, 5.45361725e+02,
1.84471257e+02, 7.99520893e+02, 4.40335942e+02, 6.93721148e+02,
7.28053940e+02, 2.21926825e+02, 2.61556126e+02, 1.65237017e+02,
5.71202456e+02, 1.09426097e+02, 3.23701588e+02, 3.19474690e+02,
2.40385568e+02, 1.78635264e+02, 1.19881449e+03, 2.63517743e+02,
4.06868552e+02, 2.66571987e+02, 2.01011583e+02, 1.37241110e+03,
6.67508730e+02, 8.01605148e+03, 2.32136932e+02, 1.59438339e+03,
1.05395523e+03, 2.28668902e+03, 1.35765084e+03, 5.24799053e+02,
4.93518719e+02, 6.90747069e+02, 4.89414748e+02, 1.25429843e+03,
2.20727361e+03, 5.46678073e+03, 1.40921377e+03, 2.64497075e+02,
3.99558627e+03, 2.56225571e+02, 1.32036801e+02, 1.03610586e+03,
1.02781888e+02, 4.42497604e+02, 1.81710343e+03, 7.45241154e+02,
2.92215226e+02, 1.93830572e+02, 8.00767434e+02, 3.79451176e+03,
4.34868956e+02, 8.32340317e+02, 2.10793287e+03, 5.52735286e+03,
1.48117020e+04, 3.11953075e+03, 2.12032186e+02, 2.03660542e+03,
5.67091277e+03, 5.36020601e+04, 3.68926783e+03, 1.70175266e+05,
1.91436414e+03, 4.04042977e+03, 2.89062509e+03, 1.64153158e+03,
2.64551029e+03, 6.91903387e+04, 3.50694462e+03, 3.13186643e+04,
5.84675136e+03, 1.10803981e+03, 2.12842765e+05, 4.19174300e+02,
4.27329763e+02, 2.77406889e+03, 2.47314709e+03, 4.91004812e+04])}
[12]:
events = SimEvents.load_from_h5("h5_test.hdf5")
[13]:
events.period("IC86_II").keys()
[13]:
dict_keys(['true_energy', 'reco_energy', 'arrival_energy', 'ang_err', 'ra', 'dec', 'source_label'])
[14]:
len(events)
[14]:
1
[15]:
"""
for i in [1.5, 2.0, 2.5, 3.0, 3.5, 3.7]:
norm_energy = 1e5 # Energy of normalisation in units of GeV
min_energy = 1e2 # GeV
max_energy = 1e8 # GeV
phi_solver = PhiSolver(aeff, norm_energy, min_energy, max_energy,
time=1, min_cosz=-1, max_cosz=1)
phi_norm = phi_solver(Nnu=2000,
dec=30, # degrees
index=i) # spectral index
phi_norm # GeV^-1 cm^-2 s^-1
point_power_law = PowerLawFlux(phi_norm, norm_energy, i,
min_energy, max_energy)
point_source = PointSource(point_power_law, z=0., coord=(np.pi, np.deg2rad(30)))
sources = [point_source]
simulator = Simulator(sources, detector)
simulator.time = 1 # year
# Run simulation
simulator.run(show_progress=True, seed=42)
simulator.save(f"data/sim_output_{i:.1f}.h5")
"""
[15]:
'\nfor i in [1.5, 2.0, 2.5, 3.0, 3.5, 3.7]:\n norm_energy = 1e5 # Energy of normalisation in units of GeV\n min_energy = 1e2 # GeV\n max_energy = 1e8 # GeV\n phi_solver = PhiSolver(aeff, norm_energy, min_energy, max_energy, \n time=1, min_cosz=-1, max_cosz=1)\n phi_norm = phi_solver(Nnu=2000, \n dec=30, # degrees\n index=i) # spectral index\n phi_norm # GeV^-1 cm^-2 s^-1\n point_power_law = PowerLawFlux(phi_norm, norm_energy, i, \n min_energy, max_energy)\n point_source = PointSource(point_power_law, z=0., coord=(np.pi, np.deg2rad(30)))\n sources = [point_source]\n simulator = Simulator(sources, detector)\n simulator.time = 1 # year\n\n # Run simulation\n simulator.run(show_progress=True, seed=42)\n simulator.save(f"data/sim_output_{i:.1f}.h5")\n'
[16]:
# Plot energies
bins = np.geomspace(1e2, max_energy)
fig, ax = plt.subplots()
ax.hist(events.true_energy["IC86_II"], bins=bins, alpha=0.7, label="E_true")
ax.hist(events.reco_energy["IC86_II"], bins=bins, alpha=0.7, label="E_reco")
ax.set_xscale("log")
ax.set_yscale("log")
ax.set_xlabel("E [GeV]")
ax.legend()
[16]:
<matplotlib.legend.Legend at 0x7f6c93e82d60>
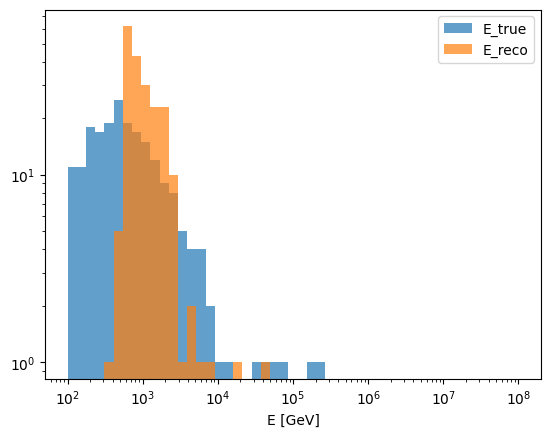
[17]:
# Plot directions
ps_sel = events.source_label["IC86_II"] == 1
fig, ax = plt.subplots(subplot_kw={"projection": "aitoff"})
fig.set_size_inches((12, 7))
circles = []
for r, d, a in zip(
events.ra["IC86_II"][~ps_sel],
events.dec["IC86_II"][~ps_sel],
events.ang_err["IC86_II"][~ps_sel],
):
circle = Circle((r - np.pi, d), radius=np.deg2rad(a))
circles.append(circle)
df_nu = PatchCollection(circles)
circles = []
for r, d, a in zip(
events.ra["IC86_II"][ps_sel],
events.dec["IC86_II"][ps_sel],
events.ang_err["IC86_II"][ps_sel],
):
circle = Circle((r - np.pi, d), radius=np.deg2rad(a))
circles.append(circle)
ps_nu = PatchCollection(circles, color="r")
ax.add_collection(df_nu)
ax.add_collection(ps_nu)
ax.grid()
fig.savefig("example_simulation.png", dpi=150)
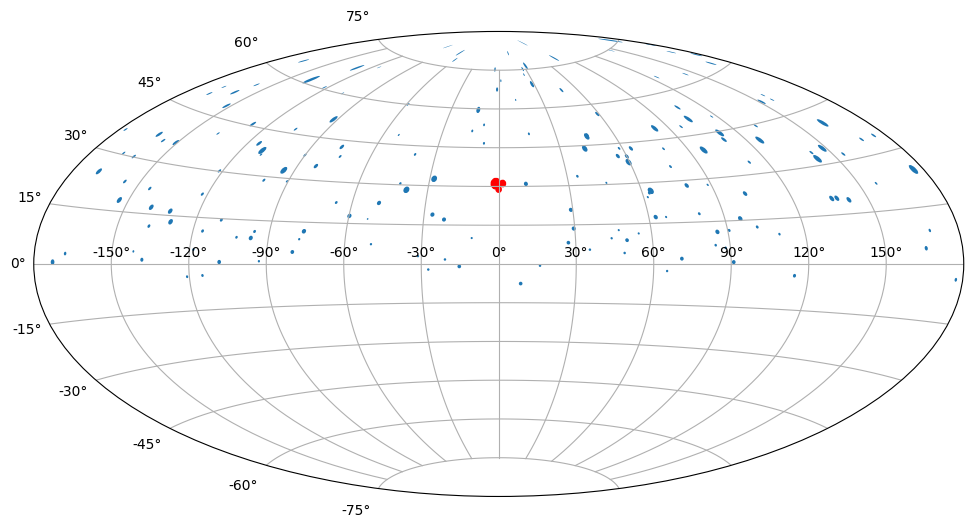
Time dependent simulation
We can simulate an observation campaign spanning multiple data periods of IceCube through a “meta class” TimeDependentSimulator
:
[18]:
tsim = TimeDependentSimulator(["IC86_I", "IC86_II"], sources)
/opt/hostedtoolcache/Python/3.9.18/x64/lib/python3.9/site-packages/icecube_tools/detector/r2021.py:89: RuntimeWarning: divide by zero encountered in log10
self.dataset[:, 6:-1] = np.log10(self.dataset[:, 6:-1])
Empty true energy bins at: [(0, 0), (1, 0)]
Need to set simulation times, defaults to 1 year each.
We need to set simulation times for all periods. Since for past periods the simulation time shouldn’t be larger than the actual observation time (that is time span - down time of the detector) we need to take care, or rather, we let the class Uptime
take care:
[19]:
from icecube_tools.utils.data import Uptime
It lets us calculate the actual observation time through, e.g. IC86_II, vs the time covered:
[20]:
uptime = Uptime("IC86_I", "IC86_II")
uptime._time_obs("IC86_II"), uptime.time_span()["IC86_II"]
[20]:
(0.9089039325939252, 1.0157235341273203)
We can further define a start and end time of an observation and let it calculate the observation time in each period. Viable possible options are - start and end time in MJD - start time in MJD and duration in years - end time in MJD and duration in years
If the start time is before the earliest period (IC_40), the start time will be set to the earliest possible date.
If the end time is past the last period (IC86_II), then we get an according message and simulate into the future.
We can of course bypass this time setting and operate directly on the instances of Simulator, for example if we’d want to build up large statistics for subsequent likelihood analysis.
[21]:
times = uptime.find_obs_time(start=55869, duration=3)
times
[21]:
{'IC86_I': 0.4798785512080253, 'IC86_II': 2.425230588331747}
The returned dictionary can be used to set the simulation times for an instance of TimeDependentSimulator
:
[22]:
tsim.time = times
The simulation is started by calling run()
, results can be saved by save(file_prefix)
, with the filename being {file_prefix}_{p}.h5
with period p
.
[23]:
tsim.run(show_progress=True)
- Sampling: 0%| | 0/94 [00:00<?, ?it/s]
</pre>
- Sampling: 0%| | 0/94 [00:00<?, ?it/s]
end{sphinxVerbatim}
Sampling: 0%| | 0/94 [00:00<?, ?it/s]
- Sampling: 13%|█▎ | 12/94 [00:00<00:00, 119.38it/s]
</pre>
- Sampling: 13%|█▎ | 12/94 [00:00<00:00, 119.38it/s]
end{sphinxVerbatim}
Sampling: 13%|█▎ | 12/94 [00:00<00:00, 119.38it/s]
- Sampling: 32%|███▏ | 30/94 [00:00<00:00, 145.36it/s]
</pre>
- Sampling: 32%|███▏ | 30/94 [00:00<00:00, 145.36it/s]
end{sphinxVerbatim}
Sampling: 32%|███▏ | 30/94 [00:00<00:00, 145.36it/s]
- Sampling: 63%|██████▎ | 59/94 [00:00<00:00, 205.05it/s]
</pre>
- Sampling: 63%|██████▎ | 59/94 [00:00<00:00, 205.05it/s]
end{sphinxVerbatim}
Sampling: 63%|██████▎ | 59/94 [00:00<00:00, 205.05it/s]
- Sampling: 100%|██████████| 94/94 [00:00<00:00, 259.77it/s]
</pre>
- Sampling: 100%|██████████| 94/94 [00:00<00:00, 259.77it/s]
end{sphinxVerbatim}
Sampling: 100%|██████████| 94/94 [00:00<00:00, 259.77it/s]
- Sampling: 100%|██████████| 94/94 [00:00<00:00, 161.49it/s]
</pre>
- Sampling: 100%|██████████| 94/94 [00:00<00:00, 161.49it/s]
end{sphinxVerbatim}
Sampling: 100%|██████████| 94/94 [00:00<00:00, 161.49it/s]
- Sampling: 0%| | 0/486 [00:00<?, ?it/s]
</pre>
- Sampling: 0%| | 0/486 [00:00<?, ?it/s]
end{sphinxVerbatim}
Sampling: 0%| | 0/486 [00:00<?, ?it/s]
- Sampling: 8%|▊ | 41/486 [00:00<00:01, 336.40it/s]
</pre>
- Sampling: 8%|▊ | 41/486 [00:00<00:01, 336.40it/s]
end{sphinxVerbatim}
Sampling: 8%|▊ | 41/486 [00:00<00:01, 336.40it/s]
- Sampling: 16%|█▋ | 80/486 [00:00<00:01, 340.89it/s]
</pre>
- Sampling: 16%|█▋ | 80/486 [00:00<00:01, 340.89it/s]
end{sphinxVerbatim}
Sampling: 16%|█▋ | 80/486 [00:00<00:01, 340.89it/s]
- Sampling: 34%|███▍ | 166/486 [00:00<00:00, 550.81it/s]
</pre>
- Sampling: 34%|███▍ | 166/486 [00:00<00:00, 550.81it/s]
end{sphinxVerbatim}
Sampling: 34%|███▍ | 166/486 [00:00<00:00, 550.81it/s]
- Sampling: 49%|████▉ | 237/486 [00:00<00:00, 579.48it/s]
</pre>
- Sampling: 49%|████▉ | 237/486 [00:00<00:00, 579.48it/s]
end{sphinxVerbatim}
Sampling: 49%|████▉ | 237/486 [00:00<00:00, 579.48it/s]
- Sampling: 67%|██████▋ | 324/486 [00:00<00:00, 631.17it/s]
</pre>
- Sampling: 67%|██████▋ | 324/486 [00:00<00:00, 631.17it/s]
end{sphinxVerbatim}
Sampling: 67%|██████▋ | 324/486 [00:00<00:00, 631.17it/s]
- Sampling: 100%|██████████| 486/486 [00:01<00:00, 426.44it/s]
</pre>
- Sampling: 100%|██████████| 486/486 [00:01<00:00, 426.44it/s]
end{sphinxVerbatim}
Sampling: 100%|██████████| 486/486 [00:01<00:00, 426.44it/s]
[24]:
tsim._true_energy
[24]:
{'IC86_I': array([ 188.64565779, 2838.69103169, 367.03045702, 302.86551638,
4041.54858527, 104.56850236, 522.75467614, 426.24773827,
484.33570281, 914.37828968, 284.48042369, 385.77928756,
305.09653451, 512.22894559, 4033.68565151, 827.09079631,
1059.19680016, 345.38516511, 685.74336857, 278.77527675,
117.99690533, 110.32573334, 1802.52451934, 778.4410995 ,
161.31934235, 423.99437872, 41263.84753565, 570.86216909,
620.64780466, 597.79735352, 957.76179785, 694.11484137,
184.95209007, 309.86561253, 116.95305688, 501.14146051,
2932.71252223, 147.56917441, 1447.49260734, 457.06727436,
404.93199983, 750.86366987, 247.89242896, 127.09485487,
305.99211461, 129.49500423, 182.51651381, 1066.13998262,
261.69269187, 641.28701347, 650.12902535, 164.81450688,
163.53010379, 1029.08167752, 450.77505447, 277.44953718,
670.65363765, 314.97978206, 258.4858178 , 1059.98925543,
1220.68227825, 530.30995951, 1646.07310385, 3044.83223386,
827.79837771, 560.69996491, 275.41742031, 302.21602598,
148.95358241, 1512.00707072, 719.0062802 , 1109.60521722,
189.48054451, 408.06317322, 1021.19858075, 1213.95201094,
694.71062439, 2079.26819735, 735.0578868 , 2704.20119544,
1003.56221726, 167.2369306 , 25744.48204983, 36546.59337264,
9074.55922984, 7479.85693133, 1614.45914584, 7085.30665897,
13767.96493166, 774.95742683, 62746.83123524, 3962.12918635,
6214.93062513, 45855.05913768]),
'IC86_II': array([1.59323877e+02, 3.81741447e+02, 9.62988295e+02, 4.00984486e+02,
2.10171395e+02, 3.21767941e+02, 1.06217717e+02, 2.00735487e+02,
1.16268257e+03, 2.97997709e+02, 3.24316790e+02, 4.02011083e+02,
2.91842080e+02, 2.11567051e+02, 4.80318064e+02, 1.56521406e+03,
6.86068788e+02, 2.59379206e+02, 1.00144005e+03, 1.14476618e+03,
1.65491687e+02, 3.81214108e+02, 8.24709899e+02, 1.13668495e+02,
2.51607529e+03, 3.33544877e+02, 1.11866026e+03, 3.00907799e+03,
6.03595872e+02, 5.17836858e+02, 2.03615617e+02, 1.85914114e+03,
6.58233916e+02, 6.86156668e+02, 1.49792233e+03, 6.48014004e+02,
5.14037912e+03, 7.77520524e+02, 4.85683512e+02, 3.14375554e+02,
2.75417420e+02, 1.02675601e+03, 2.08081656e+02, 9.88174506e+02,
1.96204729e+02, 2.34087613e+03, 1.00397823e+02, 2.64376290e+03,
6.19462865e+02, 5.22237147e+03, 2.52220227e+03, 1.13891278e+02,
1.00119781e+03, 2.59534732e+02, 1.30973494e+03, 3.00089430e+02,
1.04310789e+04, 3.04320259e+02, 1.23104678e+02, 1.81779976e+02,
1.70308710e+02, 5.65099493e+03, 1.04131081e+03, 3.27005845e+02,
3.30094188e+02, 6.76590957e+02, 1.31029121e+03, 2.72670591e+02,
4.20932988e+02, 1.04847667e+03, 2.36959681e+02, 1.18089792e+03,
1.26975274e+02, 1.92502476e+02, 7.08225183e+02, 3.25594625e+03,
1.55067439e+02, 1.65966191e+02, 4.66090336e+03, 7.08134640e+02,
2.56326181e+02, 3.79472395e+02, 3.63268623e+03, 2.76467422e+02,
2.53183177e+02, 1.01390798e+03, 5.76267020e+02, 3.20991352e+02,
1.51715603e+03, 2.48782213e+03, 4.62203222e+02, 1.74086568e+03,
1.84430970e+02, 2.11208941e+02, 2.68547399e+02, 1.61062819e+02,
7.16580758e+02, 4.09451456e+02, 2.39295424e+02, 1.48663078e+02,
1.58508336e+03, 5.06173047e+02, 1.36268559e+02, 9.72825356e+02,
2.17018557e+02, 1.46281094e+02, 2.43029725e+03, 1.27456647e+02,
6.69857184e+02, 2.19274186e+03, 2.05916832e+02, 2.08702107e+02,
1.84054099e+02, 2.86537156e+02, 3.18230846e+02, 7.52644531e+02,
1.95879084e+02, 1.25797580e+02, 7.17485580e+02, 1.76597855e+02,
1.20473330e+02, 2.11543378e+02, 8.28461622e+02, 5.21143844e+02,
2.04348900e+02, 1.62453212e+03, 1.61010505e+02, 2.00665845e+02,
5.69136591e+02, 5.89314850e+02, 5.37238154e+02, 4.57058975e+02,
3.56278995e+02, 2.02819324e+02, 6.90046169e+02, 3.18419745e+02,
2.57497610e+03, 4.65477835e+02, 2.18589326e+02, 1.35031348e+02,
2.87458044e+02, 3.78701103e+02, 7.81224555e+02, 1.68579440e+03,
2.86911716e+02, 4.10576212e+02, 2.35502615e+03, 1.71309128e+02,
1.70977453e+02, 2.87196258e+03, 5.91595330e+02, 4.30673371e+02,
1.94455667e+02, 5.81550700e+02, 1.95911854e+02, 5.55415973e+02,
2.13914938e+03, 2.31143364e+02, 5.44317658e+02, 1.69052361e+02,
6.46180994e+02, 3.07199342e+02, 1.99936534e+02, 3.45145882e+03,
3.56730128e+02, 2.97933686e+02, 3.94871752e+02, 1.17595432e+03,
4.08754869e+02, 1.69300530e+02, 1.19544407e+03, 9.93151450e+02,
1.60601584e+02, 1.01125877e+02, 2.68149491e+02, 2.10554685e+02,
8.59184686e+02, 2.72938083e+03, 1.83171080e+02, 6.88621681e+02,
5.07962061e+02, 1.00341324e+02, 4.65240954e+02, 4.37154394e+02,
1.05253682e+03, 1.05801366e+02, 2.88994246e+02, 1.59595964e+02,
1.05819004e+02, 1.01443459e+02, 3.65771614e+02, 1.66373182e+03,
3.36368321e+02, 2.64177898e+02, 4.21356569e+02, 3.09120445e+02,
8.52862535e+02, 1.00022660e+02, 6.33085657e+02, 1.61481844e+02,
2.45103583e+02, 1.71871600e+02, 3.32646324e+02, 2.92352233e+02,
3.94967947e+02, 1.98202738e+02, 1.60268481e+03, 1.99672905e+02,
1.77502278e+02, 9.85266144e+02, 1.98430635e+02, 1.74548624e+02,
2.00715075e+02, 2.81113996e+02, 1.41507422e+03, 1.80277767e+02,
8.36705736e+02, 1.47302146e+04, 8.16556108e+02, 2.70626805e+04,
1.24749765e+02, 2.79056575e+03, 6.35251197e+02, 5.48543169e+02,
2.84294944e+02, 4.40133787e+02, 1.15995704e+03, 3.33754058e+02,
1.55280403e+02, 2.90184603e+02, 2.62103955e+02, 1.20912549e+03,
6.03895622e+02, 1.20160550e+02, 2.87523081e+02, 2.87401921e+02,
1.93523631e+02, 1.41669227e+04, 6.83148406e+02, 1.65867448e+03,
1.61241233e+02, 1.93227375e+02, 5.67551749e+02, 1.22327132e+02,
3.17743442e+02, 3.05186594e+03, 5.71931011e+02, 4.10354388e+02,
4.37065609e+03, 2.55669992e+02, 3.15213042e+02, 1.65691721e+03,
1.34740974e+03, 7.64174973e+02, 5.81053915e+02, 4.32915092e+02,
4.42082343e+02, 3.23578053e+02, 1.64419896e+03, 2.95766511e+03,
1.38076551e+02, 1.71473926e+02, 2.66245568e+02, 1.65228394e+02,
3.33104086e+02, 2.88050234e+02, 1.59485879e+02, 3.48468644e+02,
1.67362854e+02, 6.59553509e+02, 9.28991248e+02, 1.62546281e+02,
1.95295819e+03, 5.24397041e+02, 3.37022112e+03, 1.30944198e+03,
9.77883749e+02, 7.12872851e+02, 3.03904344e+02, 1.80592808e+02,
2.81035498e+02, 3.07439996e+02, 1.06756266e+02, 5.50437566e+02,
2.75635823e+02, 2.23050735e+03, 1.39512039e+02, 2.11440957e+02,
2.68858330e+03, 2.80172757e+03, 5.21941566e+02, 1.81603238e+02,
4.01061601e+02, 4.79606634e+02, 6.34469320e+02, 1.27028920e+02,
3.03817155e+02, 5.04741898e+02, 3.65726877e+02, 1.27984240e+02,
6.30144157e+03, 5.22191489e+02, 4.13133477e+03, 1.38636473e+03,
5.96406789e+02, 1.34745494e+03, 1.31666007e+03, 1.49321928e+03,
1.31702146e+02, 1.94695018e+03, 1.06928738e+03, 4.07766477e+02,
3.40967608e+02, 2.66100378e+02, 2.17126630e+02, 1.86824809e+03,
3.29899279e+02, 3.67212432e+02, 2.02166956e+03, 1.05524348e+02,
4.83806758e+02, 1.83378482e+03, 6.36930795e+02, 3.26616101e+02,
8.73763802e+02, 2.27249068e+02, 4.32984613e+02, 1.17545591e+02,
4.87985937e+02, 2.76802242e+02, 1.10754785e+03, 1.59159598e+02,
2.93359310e+03, 1.96010409e+02, 2.52804192e+02, 2.77211875e+03,
2.99783738e+02, 4.74852374e+03, 4.16316265e+02, 2.64004011e+02,
1.39560926e+02, 1.12513167e+03, 2.66168323e+02, 1.61090115e+02,
5.60781477e+02, 5.52376781e+02, 2.56660762e+02, 4.39178640e+02,
5.42799974e+02, 1.22050658e+02, 1.24194903e+02, 1.24724404e+02,
7.79928900e+02, 2.09004211e+02, 2.87611626e+02, 4.03879687e+02,
1.80952904e+02, 1.11174699e+03, 1.65020518e+02, 4.10336023e+02,
7.50560904e+02, 3.35198534e+02, 2.77981008e+02, 2.54082327e+02,
5.12495665e+03, 5.86687599e+02, 1.71768138e+02, 1.60723964e+02,
5.10855057e+02, 4.45876421e+02, 3.32444285e+02, 7.20620523e+02,
1.63644529e+02, 2.98748728e+02, 3.81859842e+03, 3.47348490e+03,
1.47209105e+02, 1.34350764e+03, 1.29051489e+03, 1.18603003e+03,
3.41066716e+02, 2.44862561e+02, 5.37757712e+02, 2.16532664e+02,
4.44456477e+02, 1.04562480e+02, 1.97884135e+02, 8.26339848e+02,
4.16001867e+02, 5.22605718e+02, 1.77799442e+02, 1.68338806e+02,
3.59610298e+02, 8.28780294e+02, 1.59806658e+02, 2.89493926e+02,
4.29905711e+02, 4.33281022e+02, 1.87519178e+02, 1.18919877e+02,
3.52039218e+02, 4.26345419e+02, 8.96753237e+02, 3.97902755e+03,
1.03125251e+03, 5.76438458e+02, 2.44382158e+02, 3.50007828e+02,
2.67993277e+03, 1.05434487e+02, 3.26808970e+02, 4.22104029e+03,
1.88828728e+02, 4.25572849e+02, 6.67453662e+02, 4.79331170e+02,
6.49053688e+03, 2.75190191e+03, 4.51860437e+03, 1.91436026e+03,
2.94656923e+04, 3.38965650e+03, 4.44423912e+04, 9.21759593e+03,
7.08386015e+02, 4.83798920e+03, 7.93037927e+02, 1.40404536e+03,
1.83008398e+04, 4.52013589e+03, 5.10777850e+03, 1.99681363e+03,
1.94343103e+04, 6.51554728e+03, 2.23786498e+03, 5.51482263e+03,
5.74379966e+03, 2.26575784e+03, 2.57270546e+02, 5.14097569e+05,
1.20200138e+03, 7.56704756e+02, 4.49593609e+03, 1.98982493e+04,
6.02619632e+02, 5.33331582e+03, 1.59782001e+04, 5.56558803e+03,
5.51724489e+02, 1.85534451e+03, 3.87972065e+04, 2.67827236e+03,
2.90170244e+04, 4.16269376e+03, 1.65323263e+03, 1.49620876e+04,
3.76416730e+03, 4.48742658e+03, 3.10969459e+03, 8.98310040e+02,
7.35208900e+02, 3.09801595e+02, 6.27921222e+03, 2.61891309e+05,
1.39088312e+05, 5.68326225e+04, 1.83258433e+04, 4.32451022e+02,
1.43223339e+04, 3.39705494e+02, 1.07858042e+04, 2.92548784e+04,
5.52543959e+04, 1.74058099e+03, 4.23443919e+03, 4.40734078e+02,
1.52783232e+05, 2.42687594e+04, 1.51033840e+04, 1.25521981e+04,
5.45110292e+03, 1.26967892e+04, 1.04383107e+05, 1.23498668e+04,
1.89120940e+03, 1.06750211e+03])}
[25]:
tsim.write_to_h5("multi_test.hdf5", sources)
[26]:
tsim.arrival_energy
[26]:
{'IC86_I': array([ 188.64565779, 2838.69103169, 367.03045702, 302.86551638,
4041.54858527, 104.56850236, 522.75467614, 426.24773827,
484.33570281, 914.37828968, 284.48042369, 385.77928756,
305.09653451, 512.22894559, 4033.68565151, 827.09079631,
1059.19680016, 345.38516511, 685.74336857, 278.77527675,
117.99690533, 110.32573334, 1802.52451934, 778.4410995 ,
161.31934235, 423.99437872, 41263.84753565, 570.86216909,
620.64780466, 597.79735352, 957.76179785, 694.11484137,
184.95209007, 309.86561253, 116.95305688, 501.14146051,
2932.71252223, 147.56917441, 1447.49260734, 457.06727436,
404.93199983, 750.86366987, 247.89242896, 127.09485487,
305.99211461, 129.49500423, 182.51651381, 1066.13998262,
261.69269187, 641.28701347, 650.12902535, 164.81450688,
163.53010379, 1029.08167752, 450.77505447, 277.44953718,
670.65363765, 314.97978206, 258.4858178 , 1059.98925543,
1220.68227825, 530.30995951, 1646.07310385, 3044.83223386,
827.79837771, 560.69996491, 275.41742031, 302.21602598,
148.95358241, 1512.00707072, 719.0062802 , 1109.60521722,
189.48054451, 408.06317322, 1021.19858075, 1213.95201094,
694.71062439, 2079.26819735, 735.0578868 , 2704.20119544,
1003.56221726, 167.2369306 , 25744.48204983, 36546.59337264,
9074.55922984, 7479.85693133, 1614.45914584, 7085.30665897,
13767.96493166, 774.95742683, 62746.83123524, 3962.12918635,
6214.93062513, 45855.05913768]),
'IC86_II': array([1.59323877e+02, 3.81741447e+02, 9.62988295e+02, 4.00984486e+02,
2.10171395e+02, 3.21767941e+02, 1.06217717e+02, 2.00735487e+02,
1.16268257e+03, 2.97997709e+02, 3.24316790e+02, 4.02011083e+02,
2.91842080e+02, 2.11567051e+02, 4.80318064e+02, 1.56521406e+03,
6.86068788e+02, 2.59379206e+02, 1.00144005e+03, 1.14476618e+03,
1.65491687e+02, 3.81214108e+02, 8.24709899e+02, 1.13668495e+02,
2.51607529e+03, 3.33544877e+02, 1.11866026e+03, 3.00907799e+03,
6.03595872e+02, 5.17836858e+02, 2.03615617e+02, 1.85914114e+03,
6.58233916e+02, 6.86156668e+02, 1.49792233e+03, 6.48014004e+02,
5.14037912e+03, 7.77520524e+02, 4.85683512e+02, 3.14375554e+02,
2.75417420e+02, 1.02675601e+03, 2.08081656e+02, 9.88174506e+02,
1.96204729e+02, 2.34087613e+03, 1.00397823e+02, 2.64376290e+03,
6.19462865e+02, 5.22237147e+03, 2.52220227e+03, 1.13891278e+02,
1.00119781e+03, 2.59534732e+02, 1.30973494e+03, 3.00089430e+02,
1.04310789e+04, 3.04320259e+02, 1.23104678e+02, 1.81779976e+02,
1.70308710e+02, 5.65099493e+03, 1.04131081e+03, 3.27005845e+02,
3.30094188e+02, 6.76590957e+02, 1.31029121e+03, 2.72670591e+02,
4.20932988e+02, 1.04847667e+03, 2.36959681e+02, 1.18089792e+03,
1.26975274e+02, 1.92502476e+02, 7.08225183e+02, 3.25594625e+03,
1.55067439e+02, 1.65966191e+02, 4.66090336e+03, 7.08134640e+02,
2.56326181e+02, 3.79472395e+02, 3.63268623e+03, 2.76467422e+02,
2.53183177e+02, 1.01390798e+03, 5.76267020e+02, 3.20991352e+02,
1.51715603e+03, 2.48782213e+03, 4.62203222e+02, 1.74086568e+03,
1.84430970e+02, 2.11208941e+02, 2.68547399e+02, 1.61062819e+02,
7.16580758e+02, 4.09451456e+02, 2.39295424e+02, 1.48663078e+02,
1.58508336e+03, 5.06173047e+02, 1.36268559e+02, 9.72825356e+02,
2.17018557e+02, 1.46281094e+02, 2.43029725e+03, 1.27456647e+02,
6.69857184e+02, 2.19274186e+03, 2.05916832e+02, 2.08702107e+02,
1.84054099e+02, 2.86537156e+02, 3.18230846e+02, 7.52644531e+02,
1.95879084e+02, 1.25797580e+02, 7.17485580e+02, 1.76597855e+02,
1.20473330e+02, 2.11543378e+02, 8.28461622e+02, 5.21143844e+02,
2.04348900e+02, 1.62453212e+03, 1.61010505e+02, 2.00665845e+02,
5.69136591e+02, 5.89314850e+02, 5.37238154e+02, 4.57058975e+02,
3.56278995e+02, 2.02819324e+02, 6.90046169e+02, 3.18419745e+02,
2.57497610e+03, 4.65477835e+02, 2.18589326e+02, 1.35031348e+02,
2.87458044e+02, 3.78701103e+02, 7.81224555e+02, 1.68579440e+03,
2.86911716e+02, 4.10576212e+02, 2.35502615e+03, 1.71309128e+02,
1.70977453e+02, 2.87196258e+03, 5.91595330e+02, 4.30673371e+02,
1.94455667e+02, 5.81550700e+02, 1.95911854e+02, 5.55415973e+02,
2.13914938e+03, 2.31143364e+02, 5.44317658e+02, 1.69052361e+02,
6.46180994e+02, 3.07199342e+02, 1.99936534e+02, 3.45145882e+03,
3.56730128e+02, 2.97933686e+02, 3.94871752e+02, 1.17595432e+03,
4.08754869e+02, 1.69300530e+02, 1.19544407e+03, 9.93151450e+02,
1.60601584e+02, 1.01125877e+02, 2.68149491e+02, 2.10554685e+02,
8.59184686e+02, 2.72938083e+03, 1.83171080e+02, 6.88621681e+02,
5.07962061e+02, 1.00341324e+02, 4.65240954e+02, 4.37154394e+02,
1.05253682e+03, 1.05801366e+02, 2.88994246e+02, 1.59595964e+02,
1.05819004e+02, 1.01443459e+02, 3.65771614e+02, 1.66373182e+03,
3.36368321e+02, 2.64177898e+02, 4.21356569e+02, 3.09120445e+02,
8.52862535e+02, 1.00022660e+02, 6.33085657e+02, 1.61481844e+02,
2.45103583e+02, 1.71871600e+02, 3.32646324e+02, 2.92352233e+02,
3.94967947e+02, 1.98202738e+02, 1.60268481e+03, 1.99672905e+02,
1.77502278e+02, 9.85266144e+02, 1.98430635e+02, 1.74548624e+02,
2.00715075e+02, 2.81113996e+02, 1.41507422e+03, 1.80277767e+02,
8.36705736e+02, 1.47302146e+04, 8.16556108e+02, 2.70626805e+04,
1.24749765e+02, 2.79056575e+03, 6.35251197e+02, 5.48543169e+02,
2.84294944e+02, 4.40133787e+02, 1.15995704e+03, 3.33754058e+02,
1.55280403e+02, 2.90184603e+02, 2.62103955e+02, 1.20912549e+03,
6.03895622e+02, 1.20160550e+02, 2.87523081e+02, 2.87401921e+02,
1.93523631e+02, 1.41669227e+04, 6.83148406e+02, 1.65867448e+03,
1.61241233e+02, 1.93227375e+02, 5.67551749e+02, 1.22327132e+02,
3.17743442e+02, 3.05186594e+03, 5.71931011e+02, 4.10354388e+02,
4.37065609e+03, 2.55669992e+02, 3.15213042e+02, 1.65691721e+03,
1.34740974e+03, 7.64174973e+02, 5.81053915e+02, 4.32915092e+02,
4.42082343e+02, 3.23578053e+02, 1.64419896e+03, 2.95766511e+03,
1.38076551e+02, 1.71473926e+02, 2.66245568e+02, 1.65228394e+02,
3.33104086e+02, 2.88050234e+02, 1.59485879e+02, 3.48468644e+02,
1.67362854e+02, 6.59553509e+02, 9.28991248e+02, 1.62546281e+02,
1.95295819e+03, 5.24397041e+02, 3.37022112e+03, 1.30944198e+03,
9.77883749e+02, 7.12872851e+02, 3.03904344e+02, 1.80592808e+02,
2.81035498e+02, 3.07439996e+02, 1.06756266e+02, 5.50437566e+02,
2.75635823e+02, 2.23050735e+03, 1.39512039e+02, 2.11440957e+02,
2.68858330e+03, 2.80172757e+03, 5.21941566e+02, 1.81603238e+02,
4.01061601e+02, 4.79606634e+02, 6.34469320e+02, 1.27028920e+02,
3.03817155e+02, 5.04741898e+02, 3.65726877e+02, 1.27984240e+02,
6.30144157e+03, 5.22191489e+02, 4.13133477e+03, 1.38636473e+03,
5.96406789e+02, 1.34745494e+03, 1.31666007e+03, 1.49321928e+03,
1.31702146e+02, 1.94695018e+03, 1.06928738e+03, 4.07766477e+02,
3.40967608e+02, 2.66100378e+02, 2.17126630e+02, 1.86824809e+03,
3.29899279e+02, 3.67212432e+02, 2.02166956e+03, 1.05524348e+02,
4.83806758e+02, 1.83378482e+03, 6.36930795e+02, 3.26616101e+02,
8.73763802e+02, 2.27249068e+02, 4.32984613e+02, 1.17545591e+02,
4.87985937e+02, 2.76802242e+02, 1.10754785e+03, 1.59159598e+02,
2.93359310e+03, 1.96010409e+02, 2.52804192e+02, 2.77211875e+03,
2.99783738e+02, 4.74852374e+03, 4.16316265e+02, 2.64004011e+02,
1.39560926e+02, 1.12513167e+03, 2.66168323e+02, 1.61090115e+02,
5.60781477e+02, 5.52376781e+02, 2.56660762e+02, 4.39178640e+02,
5.42799974e+02, 1.22050658e+02, 1.24194903e+02, 1.24724404e+02,
7.79928900e+02, 2.09004211e+02, 2.87611626e+02, 4.03879687e+02,
1.80952904e+02, 1.11174699e+03, 1.65020518e+02, 4.10336023e+02,
7.50560904e+02, 3.35198534e+02, 2.77981008e+02, 2.54082327e+02,
5.12495665e+03, 5.86687599e+02, 1.71768138e+02, 1.60723964e+02,
5.10855057e+02, 4.45876421e+02, 3.32444285e+02, 7.20620523e+02,
1.63644529e+02, 2.98748728e+02, 3.81859842e+03, 3.47348490e+03,
1.47209105e+02, 1.34350764e+03, 1.29051489e+03, 1.18603003e+03,
3.41066716e+02, 2.44862561e+02, 5.37757712e+02, 2.16532664e+02,
4.44456477e+02, 1.04562480e+02, 1.97884135e+02, 8.26339848e+02,
4.16001867e+02, 5.22605718e+02, 1.77799442e+02, 1.68338806e+02,
3.59610298e+02, 8.28780294e+02, 1.59806658e+02, 2.89493926e+02,
4.29905711e+02, 4.33281022e+02, 1.87519178e+02, 1.18919877e+02,
3.52039218e+02, 4.26345419e+02, 8.96753237e+02, 3.97902755e+03,
1.03125251e+03, 5.76438458e+02, 2.44382158e+02, 3.50007828e+02,
2.67993277e+03, 1.05434487e+02, 3.26808970e+02, 4.22104029e+03,
1.88828728e+02, 4.25572849e+02, 6.67453662e+02, 4.79331170e+02,
6.49053688e+03, 2.75190191e+03, 4.51860437e+03, 1.91436026e+03,
2.94656923e+04, 3.38965650e+03, 4.44423912e+04, 9.21759593e+03,
7.08386015e+02, 4.83798920e+03, 7.93037927e+02, 1.40404536e+03,
1.83008398e+04, 4.52013589e+03, 5.10777850e+03, 1.99681363e+03,
1.94343103e+04, 6.51554728e+03, 2.23786498e+03, 5.51482263e+03,
5.74379966e+03, 2.26575784e+03, 2.57270546e+02, 5.14097569e+05,
1.20200138e+03, 7.56704756e+02, 4.49593609e+03, 1.98982493e+04,
6.02619632e+02, 5.33331582e+03, 1.59782001e+04, 5.56558803e+03,
5.51724489e+02, 1.85534451e+03, 3.87972065e+04, 2.67827236e+03,
2.90170244e+04, 4.16269376e+03, 1.65323263e+03, 1.49620876e+04,
3.76416730e+03, 4.48742658e+03, 3.10969459e+03, 8.98310040e+02,
7.35208900e+02, 3.09801595e+02, 6.27921222e+03, 2.61891309e+05,
1.39088312e+05, 5.68326225e+04, 1.83258433e+04, 4.32451022e+02,
1.43223339e+04, 3.39705494e+02, 1.07858042e+04, 2.92548784e+04,
5.52543959e+04, 1.74058099e+03, 4.23443919e+03, 4.40734078e+02,
1.52783232e+05, 2.42687594e+04, 1.51033840e+04, 1.25521981e+04,
5.45110292e+03, 1.26967892e+04, 1.04383107e+05, 1.23498668e+04,
1.89120940e+03, 1.06750211e+03])}
[27]:
events = SimEvents.load_from_h5("multi_test.hdf5")
[28]:
events.arrival_energy
[28]:
{'IC86_I': array([ 188.64565779, 2838.69103169, 367.03045702, 302.86551638,
4041.54858527, 104.56850236, 522.75467614, 426.24773827,
484.33570281, 914.37828968, 284.48042369, 385.77928756,
305.09653451, 512.22894559, 4033.68565151, 827.09079631,
1059.19680016, 345.38516511, 685.74336857, 278.77527675,
117.99690533, 110.32573334, 1802.52451934, 778.4410995 ,
161.31934235, 423.99437872, 41263.84753565, 570.86216909,
620.64780466, 597.79735352, 957.76179785, 694.11484137,
184.95209007, 309.86561253, 116.95305688, 501.14146051,
2932.71252223, 147.56917441, 1447.49260734, 457.06727436,
404.93199983, 750.86366987, 247.89242896, 127.09485487,
305.99211461, 129.49500423, 182.51651381, 1066.13998262,
261.69269187, 641.28701347, 650.12902535, 164.81450688,
163.53010379, 1029.08167752, 450.77505447, 277.44953718,
670.65363765, 314.97978206, 258.4858178 , 1059.98925543,
1220.68227825, 530.30995951, 1646.07310385, 3044.83223386,
827.79837771, 560.69996491, 275.41742031, 302.21602598,
148.95358241, 1512.00707072, 719.0062802 , 1109.60521722,
189.48054451, 408.06317322, 1021.19858075, 1213.95201094,
694.71062439, 2079.26819735, 735.0578868 , 2704.20119544,
1003.56221726, 167.2369306 , 25744.48204983, 36546.59337264,
9074.55922984, 7479.85693133, 1614.45914584, 7085.30665897,
13767.96493166, 774.95742683, 62746.83123524, 3962.12918635,
6214.93062513, 45855.05913768]),
'IC86_II': array([1.59323877e+02, 3.81741447e+02, 9.62988295e+02, 4.00984486e+02,
2.10171395e+02, 3.21767941e+02, 1.06217717e+02, 2.00735487e+02,
1.16268257e+03, 2.97997709e+02, 3.24316790e+02, 4.02011083e+02,
2.91842080e+02, 2.11567051e+02, 4.80318064e+02, 1.56521406e+03,
6.86068788e+02, 2.59379206e+02, 1.00144005e+03, 1.14476618e+03,
1.65491687e+02, 3.81214108e+02, 8.24709899e+02, 1.13668495e+02,
2.51607529e+03, 3.33544877e+02, 1.11866026e+03, 3.00907799e+03,
6.03595872e+02, 5.17836858e+02, 2.03615617e+02, 1.85914114e+03,
6.58233916e+02, 6.86156668e+02, 1.49792233e+03, 6.48014004e+02,
5.14037912e+03, 7.77520524e+02, 4.85683512e+02, 3.14375554e+02,
2.75417420e+02, 1.02675601e+03, 2.08081656e+02, 9.88174506e+02,
1.96204729e+02, 2.34087613e+03, 1.00397823e+02, 2.64376290e+03,
6.19462865e+02, 5.22237147e+03, 2.52220227e+03, 1.13891278e+02,
1.00119781e+03, 2.59534732e+02, 1.30973494e+03, 3.00089430e+02,
1.04310789e+04, 3.04320259e+02, 1.23104678e+02, 1.81779976e+02,
1.70308710e+02, 5.65099493e+03, 1.04131081e+03, 3.27005845e+02,
3.30094188e+02, 6.76590957e+02, 1.31029121e+03, 2.72670591e+02,
4.20932988e+02, 1.04847667e+03, 2.36959681e+02, 1.18089792e+03,
1.26975274e+02, 1.92502476e+02, 7.08225183e+02, 3.25594625e+03,
1.55067439e+02, 1.65966191e+02, 4.66090336e+03, 7.08134640e+02,
2.56326181e+02, 3.79472395e+02, 3.63268623e+03, 2.76467422e+02,
2.53183177e+02, 1.01390798e+03, 5.76267020e+02, 3.20991352e+02,
1.51715603e+03, 2.48782213e+03, 4.62203222e+02, 1.74086568e+03,
1.84430970e+02, 2.11208941e+02, 2.68547399e+02, 1.61062819e+02,
7.16580758e+02, 4.09451456e+02, 2.39295424e+02, 1.48663078e+02,
1.58508336e+03, 5.06173047e+02, 1.36268559e+02, 9.72825356e+02,
2.17018557e+02, 1.46281094e+02, 2.43029725e+03, 1.27456647e+02,
6.69857184e+02, 2.19274186e+03, 2.05916832e+02, 2.08702107e+02,
1.84054099e+02, 2.86537156e+02, 3.18230846e+02, 7.52644531e+02,
1.95879084e+02, 1.25797580e+02, 7.17485580e+02, 1.76597855e+02,
1.20473330e+02, 2.11543378e+02, 8.28461622e+02, 5.21143844e+02,
2.04348900e+02, 1.62453212e+03, 1.61010505e+02, 2.00665845e+02,
5.69136591e+02, 5.89314850e+02, 5.37238154e+02, 4.57058975e+02,
3.56278995e+02, 2.02819324e+02, 6.90046169e+02, 3.18419745e+02,
2.57497610e+03, 4.65477835e+02, 2.18589326e+02, 1.35031348e+02,
2.87458044e+02, 3.78701103e+02, 7.81224555e+02, 1.68579440e+03,
2.86911716e+02, 4.10576212e+02, 2.35502615e+03, 1.71309128e+02,
1.70977453e+02, 2.87196258e+03, 5.91595330e+02, 4.30673371e+02,
1.94455667e+02, 5.81550700e+02, 1.95911854e+02, 5.55415973e+02,
2.13914938e+03, 2.31143364e+02, 5.44317658e+02, 1.69052361e+02,
6.46180994e+02, 3.07199342e+02, 1.99936534e+02, 3.45145882e+03,
3.56730128e+02, 2.97933686e+02, 3.94871752e+02, 1.17595432e+03,
4.08754869e+02, 1.69300530e+02, 1.19544407e+03, 9.93151450e+02,
1.60601584e+02, 1.01125877e+02, 2.68149491e+02, 2.10554685e+02,
8.59184686e+02, 2.72938083e+03, 1.83171080e+02, 6.88621681e+02,
5.07962061e+02, 1.00341324e+02, 4.65240954e+02, 4.37154394e+02,
1.05253682e+03, 1.05801366e+02, 2.88994246e+02, 1.59595964e+02,
1.05819004e+02, 1.01443459e+02, 3.65771614e+02, 1.66373182e+03,
3.36368321e+02, 2.64177898e+02, 4.21356569e+02, 3.09120445e+02,
8.52862535e+02, 1.00022660e+02, 6.33085657e+02, 1.61481844e+02,
2.45103583e+02, 1.71871600e+02, 3.32646324e+02, 2.92352233e+02,
3.94967947e+02, 1.98202738e+02, 1.60268481e+03, 1.99672905e+02,
1.77502278e+02, 9.85266144e+02, 1.98430635e+02, 1.74548624e+02,
2.00715075e+02, 2.81113996e+02, 1.41507422e+03, 1.80277767e+02,
8.36705736e+02, 1.47302146e+04, 8.16556108e+02, 2.70626805e+04,
1.24749765e+02, 2.79056575e+03, 6.35251197e+02, 5.48543169e+02,
2.84294944e+02, 4.40133787e+02, 1.15995704e+03, 3.33754058e+02,
1.55280403e+02, 2.90184603e+02, 2.62103955e+02, 1.20912549e+03,
6.03895622e+02, 1.20160550e+02, 2.87523081e+02, 2.87401921e+02,
1.93523631e+02, 1.41669227e+04, 6.83148406e+02, 1.65867448e+03,
1.61241233e+02, 1.93227375e+02, 5.67551749e+02, 1.22327132e+02,
3.17743442e+02, 3.05186594e+03, 5.71931011e+02, 4.10354388e+02,
4.37065609e+03, 2.55669992e+02, 3.15213042e+02, 1.65691721e+03,
1.34740974e+03, 7.64174973e+02, 5.81053915e+02, 4.32915092e+02,
4.42082343e+02, 3.23578053e+02, 1.64419896e+03, 2.95766511e+03,
1.38076551e+02, 1.71473926e+02, 2.66245568e+02, 1.65228394e+02,
3.33104086e+02, 2.88050234e+02, 1.59485879e+02, 3.48468644e+02,
1.67362854e+02, 6.59553509e+02, 9.28991248e+02, 1.62546281e+02,
1.95295819e+03, 5.24397041e+02, 3.37022112e+03, 1.30944198e+03,
9.77883749e+02, 7.12872851e+02, 3.03904344e+02, 1.80592808e+02,
2.81035498e+02, 3.07439996e+02, 1.06756266e+02, 5.50437566e+02,
2.75635823e+02, 2.23050735e+03, 1.39512039e+02, 2.11440957e+02,
2.68858330e+03, 2.80172757e+03, 5.21941566e+02, 1.81603238e+02,
4.01061601e+02, 4.79606634e+02, 6.34469320e+02, 1.27028920e+02,
3.03817155e+02, 5.04741898e+02, 3.65726877e+02, 1.27984240e+02,
6.30144157e+03, 5.22191489e+02, 4.13133477e+03, 1.38636473e+03,
5.96406789e+02, 1.34745494e+03, 1.31666007e+03, 1.49321928e+03,
1.31702146e+02, 1.94695018e+03, 1.06928738e+03, 4.07766477e+02,
3.40967608e+02, 2.66100378e+02, 2.17126630e+02, 1.86824809e+03,
3.29899279e+02, 3.67212432e+02, 2.02166956e+03, 1.05524348e+02,
4.83806758e+02, 1.83378482e+03, 6.36930795e+02, 3.26616101e+02,
8.73763802e+02, 2.27249068e+02, 4.32984613e+02, 1.17545591e+02,
4.87985937e+02, 2.76802242e+02, 1.10754785e+03, 1.59159598e+02,
2.93359310e+03, 1.96010409e+02, 2.52804192e+02, 2.77211875e+03,
2.99783738e+02, 4.74852374e+03, 4.16316265e+02, 2.64004011e+02,
1.39560926e+02, 1.12513167e+03, 2.66168323e+02, 1.61090115e+02,
5.60781477e+02, 5.52376781e+02, 2.56660762e+02, 4.39178640e+02,
5.42799974e+02, 1.22050658e+02, 1.24194903e+02, 1.24724404e+02,
7.79928900e+02, 2.09004211e+02, 2.87611626e+02, 4.03879687e+02,
1.80952904e+02, 1.11174699e+03, 1.65020518e+02, 4.10336023e+02,
7.50560904e+02, 3.35198534e+02, 2.77981008e+02, 2.54082327e+02,
5.12495665e+03, 5.86687599e+02, 1.71768138e+02, 1.60723964e+02,
5.10855057e+02, 4.45876421e+02, 3.32444285e+02, 7.20620523e+02,
1.63644529e+02, 2.98748728e+02, 3.81859842e+03, 3.47348490e+03,
1.47209105e+02, 1.34350764e+03, 1.29051489e+03, 1.18603003e+03,
3.41066716e+02, 2.44862561e+02, 5.37757712e+02, 2.16532664e+02,
4.44456477e+02, 1.04562480e+02, 1.97884135e+02, 8.26339848e+02,
4.16001867e+02, 5.22605718e+02, 1.77799442e+02, 1.68338806e+02,
3.59610298e+02, 8.28780294e+02, 1.59806658e+02, 2.89493926e+02,
4.29905711e+02, 4.33281022e+02, 1.87519178e+02, 1.18919877e+02,
3.52039218e+02, 4.26345419e+02, 8.96753237e+02, 3.97902755e+03,
1.03125251e+03, 5.76438458e+02, 2.44382158e+02, 3.50007828e+02,
2.67993277e+03, 1.05434487e+02, 3.26808970e+02, 4.22104029e+03,
1.88828728e+02, 4.25572849e+02, 6.67453662e+02, 4.79331170e+02,
6.49053688e+03, 2.75190191e+03, 4.51860437e+03, 1.91436026e+03,
2.94656923e+04, 3.38965650e+03, 4.44423912e+04, 9.21759593e+03,
7.08386015e+02, 4.83798920e+03, 7.93037927e+02, 1.40404536e+03,
1.83008398e+04, 4.52013589e+03, 5.10777850e+03, 1.99681363e+03,
1.94343103e+04, 6.51554728e+03, 2.23786498e+03, 5.51482263e+03,
5.74379966e+03, 2.26575784e+03, 2.57270546e+02, 5.14097569e+05,
1.20200138e+03, 7.56704756e+02, 4.49593609e+03, 1.98982493e+04,
6.02619632e+02, 5.33331582e+03, 1.59782001e+04, 5.56558803e+03,
5.51724489e+02, 1.85534451e+03, 3.87972065e+04, 2.67827236e+03,
2.90170244e+04, 4.16269376e+03, 1.65323263e+03, 1.49620876e+04,
3.76416730e+03, 4.48742658e+03, 3.10969459e+03, 8.98310040e+02,
7.35208900e+02, 3.09801595e+02, 6.27921222e+03, 2.61891309e+05,
1.39088312e+05, 5.68326225e+04, 1.83258433e+04, 4.32451022e+02,
1.43223339e+04, 3.39705494e+02, 1.07858042e+04, 2.92548784e+04,
5.52543959e+04, 1.74058099e+03, 4.23443919e+03, 4.40734078e+02,
1.52783232e+05, 2.42687594e+04, 1.51033840e+04, 1.25521981e+04,
5.45110292e+03, 1.26967892e+04, 1.04383107e+05, 1.23498668e+04,
1.89120940e+03, 1.06750211e+03])}
[29]:
"""
for i in [3.9]:
norm_energy = 1e5 # Energy of normalisation in units of GeV
min_energy = 1e2 # GeV
max_energy = 1e8 # GeV
phi_solver = PhiSolver(aeff, norm_energy, min_energy, max_energy,
time=1, min_cosz=-1, max_cosz=1)
phi_norm = phi_solver(Nnu=2000,
dec=30, # degrees
index=i) # spectral index
phi_norm # GeV^-1 cm^-2 s^-1
point_power_law = PowerLawFlux(phi_norm, norm_energy, i,
min_energy, max_energy)
point_source = PointSource(point_power_law, z=0., coord=(np.pi, np.deg2rad(30)))
sources = [point_source]
tsim = TimeDependentSimulator(["IC86_I", "IC86_II"], sources)
for sim in tsim.simulators.values():
sim.time = 1
tsim.run(show_progress=True)
tsim.save(f"index_{i}")
"""
[29]:
'\nfor i in [3.9]:\n norm_energy = 1e5 # Energy of normalisation in units of GeV\n min_energy = 1e2 # GeV\n max_energy = 1e8 # GeV\n phi_solver = PhiSolver(aeff, norm_energy, min_energy, max_energy, \n time=1, min_cosz=-1, max_cosz=1)\n phi_norm = phi_solver(Nnu=2000, \n dec=30, # degrees\n index=i) # spectral index\n phi_norm # GeV^-1 cm^-2 s^-1\n point_power_law = PowerLawFlux(phi_norm, norm_energy, i, \n min_energy, max_energy)\n point_source = PointSource(point_power_law, z=0., coord=(np.pi, np.deg2rad(30)))\n sources = [point_source]\n tsim = TimeDependentSimulator(["IC86_I", "IC86_II"], sources)\n for sim in tsim.simulators.values():\n sim.time = 1\n tsim.run(show_progress=True)\n tsim.save(f"index_{i}")\n'
[ ]: